Automate Google Ads Campaigns Based on Weather with OpenWeather API
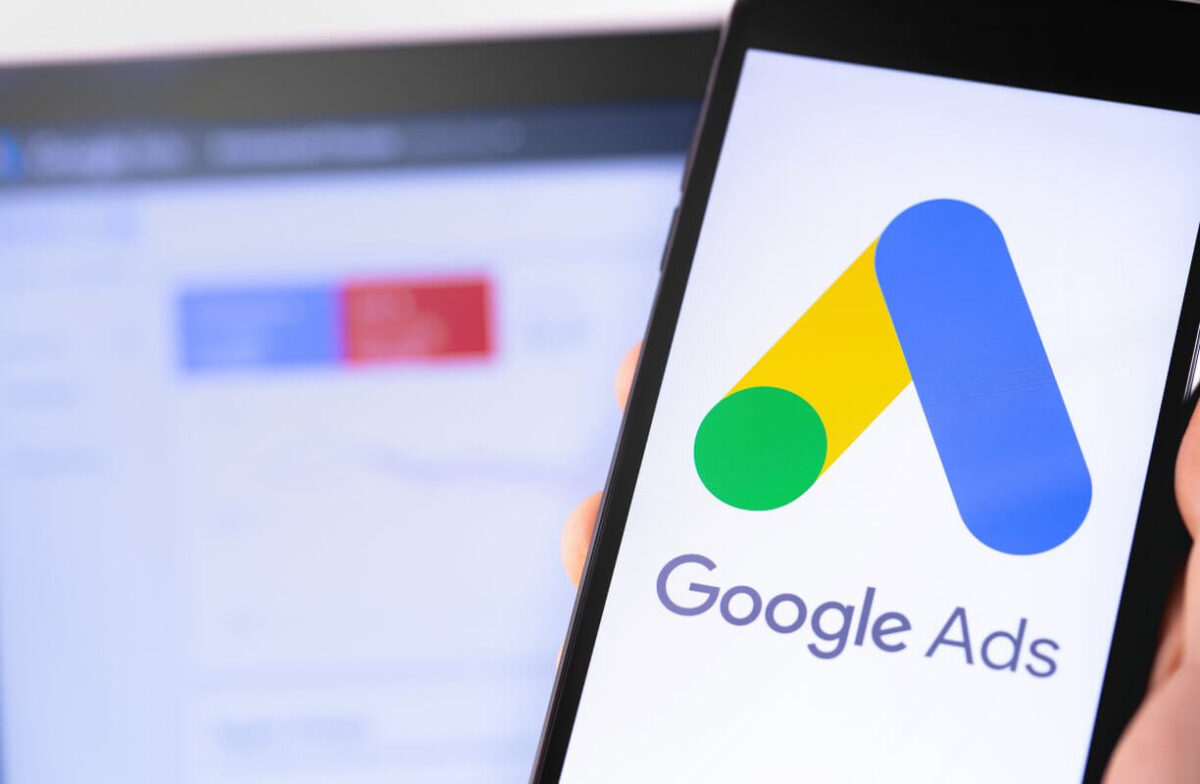
For businesses like basement waterproofing, where customer demand spikes during and after rain, automating your Google Ads campaigns based on local weather conditions can significantly optimize your ad spend. This blog post provides a step-by-step guide to creating a Google Ads script that starts and pauses your campaigns based on real-time weather data, ensuring your ads are most visible when potential customers are actively seeking your services.
Article Breakdown
Why Automate Google Ads Based on Weather?
For industries like basement waterproofing, roofing, or lawn care, weather plays a crucial role in driving demand. Ads served during or shortly after rain can reach customers exactly when they’re most in need of your services. By automating your campaigns to run only during these peak times, you ensure your budget is spent effectively, maximizing ROI.
Step 1: Get Your Location’s Latitude and Longitude
To retrieve weather data, you’ll need your location’s latitude and longitude. Here’s how to find them:
- Google Maps:
- Go to Google Maps.
- Enter your business address.
- Right-click on the location pin and select “What’s here?”
- The latitude and longitude will appear at the bottom of the screen.
Step 2: Create an Account on OpenWeather
- Sign Up:
- Visit OpenWeather and create an account.
- Get API Key:
- Once logged in, navigate to the API keys section and generate a new API key.
Step 3: Set Up a Google Sheet
You’ll use Google Sheets to store the last rain timestamp, which helps control how long your ads run after it stops raining.
- Create a Google Sheet:
- Create a new Google Sheet.
- Note down the Spreadsheet ID from the URL.
Step 4: Implement the Google Ads Script
Now, you’re ready to set up your Google Ads automation.
- Access Google Ads Scripts:
- Log in to your Google Ads account.
- Navigate to “Tools & Settings” > “Scripts” under the “Bulk Actions” section.
- Click the “+” button to create a new script.
- Copy and Paste the Script:
function main() {
var location = { lat: YOUR_LATITUDE, lon: YOUR_LONGITUDE }; // Replace with your location
var apiKey = 'YOUR_OPENWEATHER_API_KEY'; // Replace with your OpenWeather API key
var apiUrl = 'https://api.openweathermap.org/data/3.0/onecall?lat=' + location.lat + '&lon=' + location.lon + '&exclude=minutely,hourly,daily,alerts&units=metric&appid=' + apiKey;
var response = UrlFetchApp.fetch(apiUrl);
var weatherData = JSON.parse(response.getContentText());
// Check if it's currently raining
var isRaining = weatherData.current.weather.some(function(condition) {
return condition.main.toLowerCase().includes('rain');
});
// Campaign details
var campaignName = 'Rainy Day Campaign';
var campaignIterator = AdsApp.campaigns()
.withCondition("Name = '" + campaignName + "'")
.get();
// Define how many hours after rain stops the campaign should keep running
var postRainHours = 24; // Customize the duration after rain
var currentTime = new Date().getTime();
var lastRainTime = getLastRainTime();
if (isRaining) {
setLastRainTime(currentTime);
enableCampaign(campaignIterator);
} else if (currentTime - lastRainTime <= postRainHours * 60 * 60 * 1000) {
enableCampaign(campaignIterator);
} else {
pauseCampaign(campaignIterator);
}
}
// Helper function to enable the campaign
function enableCampaign(campaignIterator) {
while (campaignIterator.hasNext()) {
var campaign = campaignIterator.next();
if (!campaign.isEnabled()) {
campaign.enable();
}
}
}
// Helper function to pause the campaign
function pauseCampaign(campaignIterator) {
while (campaignIterator.hasNext()) {
var campaign = campaignIterator.next();
if (campaign.isEnabled()) {
campaign.pause();
}
}
}
// Store the last rain time in a Google Sheet
function setLastRainTime(time) {
var sheet = getSheet();
sheet.getRange('A1').setValue(time);
}
// Retrieve the last rain time from the Google Sheet
function getLastRainTime() {
var sheet = getSheet();
var storedTime = sheet.getRange('A1').getValue();
return storedTime ? parseInt(storedTime, 10) : 0;
}
// Get the Google Sheet where the last rain time is stored
function getSheet() {
var spreadsheetId = 'YOUR_SPREADSHEET_ID'; // Replace with your Google Sheet ID
var sheetName = 'LastRainTime'; // Replace with your desired sheet name
var sheet = SpreadsheetApp.openById(spreadsheetId).getSheetByName(sheetName);
if (!sheet) {
var spreadsheet = SpreadsheetApp.openById(spreadsheetId);
sheet = spreadsheet.insertSheet(sheetName);
}
return sheet;
}
- Customize the Script:
- Replace
YOUR_LATITUDE
andYOUR_LONGITUDE
with the coordinates of your business. - Replace
YOUR_OPENWEATHER_API_KEY
with the API key you obtained. - Replace
YOUR_SPREADSHEET_ID
with the ID of your Google Sheet.
- Replace
- Authorize and Test:
- Authorize the script and run a test to ensure it works as expected.
- Schedule the Script:
- Schedule the script to run every hour or more frequently to ensure real-time adjustments to your campaigns.
Conclusion
Automating your Google Ads campaigns based on weather conditions is a powerful strategy for businesses reliant on specific weather events. By following these steps, you can set up a fully automated system that ensures your ads are only running when they’re most effective, helping you maximize ROI and better serve your customers during critical times.
Implement this solution today and optimize your ad spend based on real-time weather conditions!